Studyguide for Xamarin Mobile Developer exam
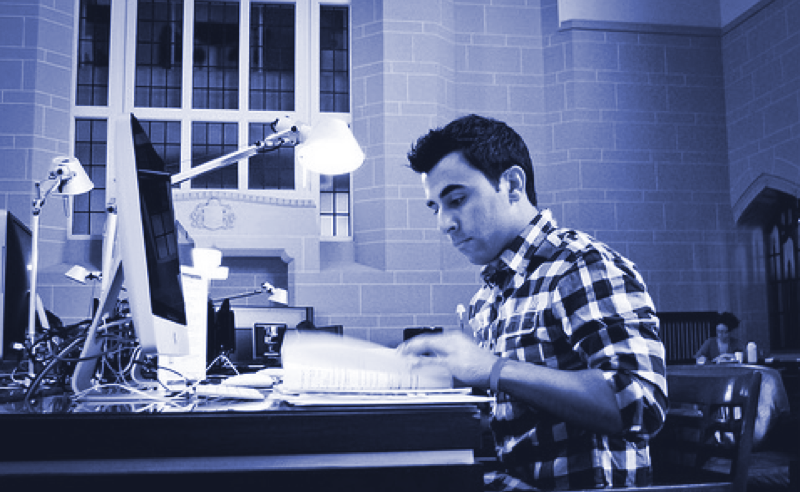
Posted on May 20, 2014
I have collected the links to additional information provided in each of the session videos. If any links are missing, please add them in the comments. The link collection can be used as a studyguide for Xamarin Mobile Developer Exam. XAM101 Intro to Mobile / Kickstart http://docs.xamarin.com/guides/cross-platform/application_fundamentals/building_cross_platform_applications/ AND101 Intro to Android with Xamarin Studio http://docs.xamarin.com/guides/android/getting_started/… Continue Reading Studyguide for Xamarin Mobile Developer exam